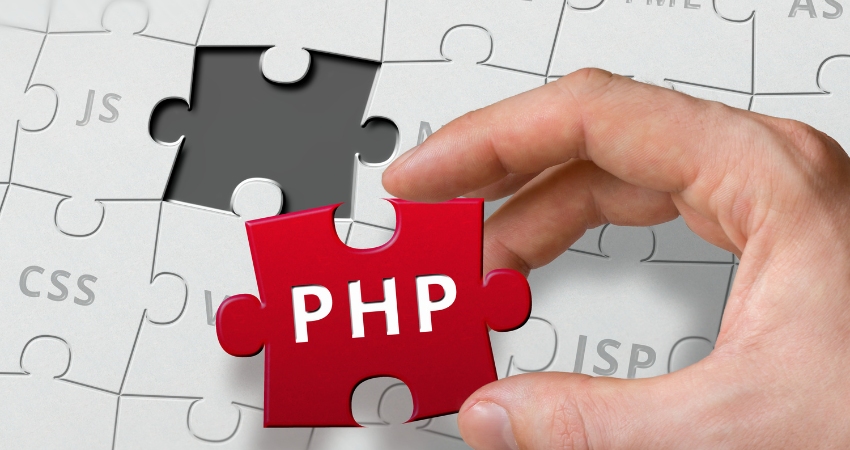
Error handling is a critical aspect of PHP development, ensuring smooth execution and graceful recovery from unforeseen issues. Exception handling provides a structured approach to manage errors, allowing developers to control and respond to exceptional situations. In this guide, we'll explore error handling techniques, exception management, and best practices in PHP.
Understanding Errors in PHP
-
Types of Errors
Syntax Errors : Detected during script compilation, preventing script execution.
Runtime Errors : Occur during script execution due to unexpected conditions. -
Error Reporting
Displaying Errors : Configuring error reporting settings in PHP.ini or runtime for debugging.
Error Types and Levels : Differentiating between notices, warnings, and fatal errors.
Basic Error Handling in PHP
-
Using die() and exit()
Immediate Termination : Halting script execution and displaying custom error messages.
-
Error Logging
Logging Errors : Configuring error logs to record errors for debugging purposes.
Error Log Formats and Locations : Specifying log formats and locations for error tracking.
Exception Handling in PHP
-
Introduction to Exceptions
Exception Class Hierarchy : Understanding the Exception class and inheritance.
Throwing Exceptions : Raising exceptions using the throw statement. -
Try-Catch Blocks
Catching Exceptions : Using try-catch blocks to handle exceptions gracefully.
Multiple Catch Blocks : Handling different types of exceptions separately within a try-catch block.
Custom Exception Handling
-
Creating Custom Exceptions
Extending Exception Class : Defining custom exception classes for specific error scenarios.
Throwing Custom Exceptions : Raising and handling custom exceptions. -
Exception Handling Strategies
Graceful Degradation : Implementing fallback mechanisms in case of exceptions.
Logging and Recovery : Logging exceptions and taking appropriate recovery actions.
Best Practices for Effective Error Management
-
Fail Fast Principle
Identifying Errors Early : Detecting errors at an early stage for faster resolution.
-
Clear Error Messages
Informative Messages : Providing descriptive error messages for effective debugging.
User-Friendly Feedback : Presenting user-friendly error messages for end-users.
Conclusion -
Error handling and exception management are crucial aspects of PHP development, ensuring robustness and reliability in applications. By implementing effective error handling strategies, developers can identify, manage, and recover from errors gracefully, enhancing the stability and user experience of PHP applications.
Adopting best practices in error handling and exception management empowers developers to create more resilient and maintainable PHP codebases.